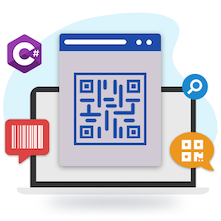
Barcodes play a crucial role in product identification, offering machine-readable representations of data. Common formats such as Code128, QR, DataMatrix, and Aztec are extensively utilized across various industries. In this guide, you’ll discover how to generate barcodes in C# and effortlessly customize their designs using the powerful Aspose.BarCode Plugins.
Table of Contents
- C# Barcode Generator API
- How to Generate a Barcode using C#
- How to Generate a QR Barcode using C#
- Customize the Appearance of a Barcode in C#
- Add Caption in Barcodes using C#
C# Barcode Generator API - Free Download
The Aspose.BarCode for .NET library is a comprehensive API designed for barcode generation and scanning, making it the ideal C# Free Barcode Generator. It allows you to create and read a variety of barcode symbologies, including:
- Code128
- Code11
- Code39
- QR
- DataMatrix
- EAN13
- EAN8
- ITF14
- PDF417
- many more.
You can download the API for free, or install it directly in your .NET application using NuGet for a C# Barcode Generator NuGet solution:
PM> Install-Package Aspose.Barcode
How to Generate a Barcode using C#
Generating a barcode with the Aspose Plugin is both straightforward and efficient. Follow these steps to create a barcode using C#, utilizing Barcode Generator C# Source Code:
- Instantiate the BarcodeGenerator class, specifying the desired barcode type and text in the constructor.
- Configure the barcode’s features, such as resolution and size.
- Generate the barcode using the BarcodeGenerator.Save(String) method.
Here’s a code sample demonstrating how to generate a barcode using Barcode C# SDK:
BarcodeGenerator generator = new BarcodeGenerator(EncodeTypes.Code128, "Aspose.BarCode"); | |
// set resolution | |
generator.Parameters.Resolution = 400; | |
// generate barcode | |
generator.Save("generate-barcode.png"); |
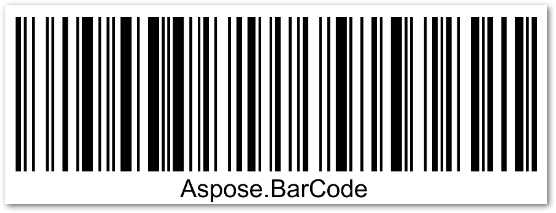
How to Generate a QR Barcode using C#
Creating a QR barcode follows a similar process to generating other barcode types. Here’s how to do it:
- Instantiate the
BarcodeGenerator
class, specifying the barcode type as EncodeTypes.QR. - Generate the barcode using the
BarcodeGenerator.Save(String)
method.
Here’s a code sample for generating a QR barcode using C#:
BarcodeGenerator generator = new BarcodeGenerator(EncodeTypes.QR, "Aspose.BarCode"); | |
// set resolution | |
generator.Parameters.Resolution = 400; | |
// generate barcode | |
generator.Save("generate-barcode.png"); |
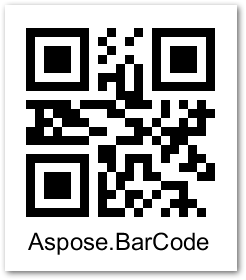
Customize the Appearance of a Barcode in C#
Customizing the appearance of a barcode is easy with the Aspose Plugin. You can modify its font, foreground color, background color, and text color. Here are the steps:
- Instantiate the
BarcodeGenerator
class. - Adjust the barcode’s appearance using the
BarcodeGenerator.Parameters
properties, such as BarcodeGenerator.Parameters.BackColor. - Generate the barcode using the
BarcodeGenerator.Save(String)
.
Here’s a code sample for generating a customized Aztec
barcode using Barcode Generator C#:
BarcodeGenerator generator = new BarcodeGenerator(EncodeTypes.Aztec, "Aspose.BarCode"); | |
// set barcode's back color | |
generator.Parameters.BackColor = System.Drawing.Color.DarkGray; | |
// set barcode's bar color | |
generator.Parameters.Barcode.BarColor = System.Drawing.Color.Orange; | |
// set border color | |
generator.Parameters.Border.Color = System.Drawing.Color.Black; | |
// set text color | |
generator.Parameters.Barcode.CodeTextParameters.Color = System.Drawing.Color.Orange; | |
// set resolution | |
generator.Parameters.Resolution = 400; | |
// generate barcode | |
generator.Save("generate-barcode.png"); |
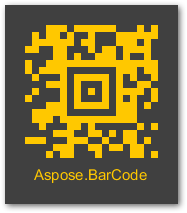
Add Caption in Barcodes using C#
Incorporating captions into barcodes can provide additional context, and the Aspose Plugin makes this process seamless. Here’s how to add a caption:
- Instantiate the
BarcodeGenerator
class. - Set the barcode’s text and type in the constructor of BarcodeGenerator.
- Utilize the
CaptionAbove
orCaptionBelow
properties to configure the caption. - Save the barcode using the
BarcodeGenerator.Save(String)
method.
Here’s a code sample for adding a caption to a barcode using Barcode Generator C#:
BarcodeGenerator generator = new BarcodeGenerator(EncodeTypes.Code128, "Aspose.BarCode"); | |
// set captions | |
generator.Parameters.CaptionAbove.Text = "The caption above."; | |
generator.Parameters.CaptionAbove.Visible = true; | |
generator.Parameters.CaptionBelow.Text = "The caption below."; | |
generator.Parameters.CaptionBelow.Visible = true; | |
// generate barcode | |
generator.Save("generate-barcode.png"); |
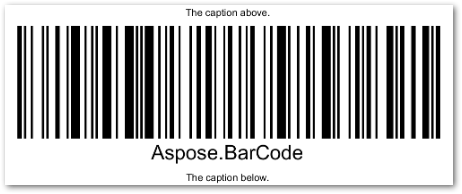
Conclusion
In this article, you learned how to programmatically generate barcodes using C#. You also explored how to customize their appearance and add captions. For more detailed information, visit the Aspose.BarCode Plugin Documentation to enhance your barcode generation capabilities with C# Barcode Generator options.
More in this category
- Aspose.BarCode - Barcode Generation and Recognition Solution
- Generate Barcodes with UTF-8 Encoding using C# .NET
- QR Code Generation in C# with Aspose.BarCode 2D Plugin
- Generate and Display Barcode Image in ASP.NET MVC Application
- Data Matrix Code Generation with Aspose.BarCode's 2D Barcode Writer Plugin