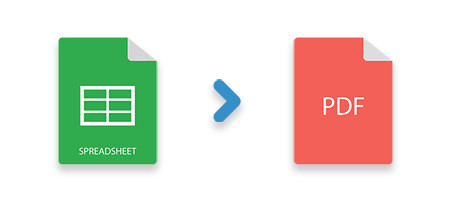
Excel files are versatile for creating and analyzing data, but converting them to PDF is often necessary for sharing or archiving purposes. In this guide, we’ll explore how to use Aspose.Cells for .NET, a reliable C# Excel to PDF converter, to programmatically convert Excel spreadsheets (XLSX/XLS) to PDF in C#. This high-performance library ensures seamless formatting and layout retention while offering advanced customization options.
Key Topics:
- C# Excel to PDF Conversion Library
- Steps to Convert an Excel File to PDF in C#
- Convert Excel XLSX to PDF in C#
- Customize PDF Compliance in Excel to PDF
- Track Conversion Progress in C#
- Get Free Access to the Plugin
C# Excel to PDF Conversion Library
Aspose.Cells for .NET is a comprehensive C# library for Excel to PDF conversion designed for robust Excel-to-PDF conversion. It supports multiple Excel formats, such as XLS, XLSX, CSV, and more, while preserving the original layout and styling.
Features:
- Converts spreadsheets to high-quality PDFs.
- Maintains the integrity of Excel formatting and layout.
- Offers advanced options for compliance, such as PDF/A.
Install via NuGet:
PM> Install-Package Aspose.Cells
Steps to Convert an Excel File to PDF in C#
Follow these simple steps to convert Excel to PDF C# applications:
- Load the Excel file using the
Workbook
class. - Save it as a PDF using the
Save
method withSaveFormat.Pdf
.
Now, let’s explore this process with a practical example.
Convert Excel XLSX to PDF in C#
Convert Excel files to PDF with just a few lines of code using Aspose.Cells for .NET:
// Instantiate the Workbook object with the Excel file
Workbook workbook = new Workbook("SampleExcel.xlsx");
// Save the document in PDF format
workbook.Save("outputPDF.pdf", SaveFormat.Pdf);
This ensures a quick and efficient Excel to PDF conversion process while retaining all formatting details from the original spreadsheet.
Customize PDF Compliance in Excel to PDF
Generate PDF/A-compliant files for long-term archiving using the PdfSaveOptions
class. PDF/A ensures compatibility and prevents issues caused by unsupported features.
// Instantiate a Workbook object and load an Excel file
Workbook workbook = new Workbook("SampleExcel.xlsx");
// Configure PDF save options for compliance
PdfSaveOptions pdfSaveOptions = new PdfSaveOptions
{
Compliance = PdfCompliance.PdfA1b
};
// Save the document in PDF/A format
workbook.Save("output-compliant.pdf", pdfSaveOptions);
This feature is ideal for industries requiring strict compliance standards.
Track Conversion Progress in C#
Monitor the progress of your Excel-to-PDF conversions using the IPageSavingCallback
interface. This allows you to take actions, such as excluding specific pages from the output.
// Load the workbook
Workbook workbook = new Workbook("PagesBook1.xlsx");
// Define PDF save options and a custom callback
PdfSaveOptions pdfSaveOptions = new PdfSaveOptions
{
PageSavingCallback = new CustomPageSavingCallback()
};
workbook.Save("TrackedConversion.pdf", pdfSaveOptions);
// Custom callback implementation
public class CustomPageSavingCallback : IPageSavingCallback
{
public void PageStartSaving(PageStartSavingArgs args)
{
Console.WriteLine($"Saving page {args.PageIndex + 1} of {args.PageCount}...");
if (args.PageIndex < 1) args.IsToOutput = false; // Exclude first page
}
public void PageEndSaving(PageEndSavingArgs args)
{
Console.WriteLine($"Finished saving page {args.PageIndex + 1}.");
}
}
Get Free Access to the Plugin
You can evaluate the Aspose.Cells PDF Converter Plugin without limitations by requesting a free trial. This allows you to explore the full capabilities of this reliable C# Excel to PDF converter before purchase.
Conclusion
Converting Excel spreadsheets to PDF is a common requirement for document sharing, archiving, and compliance. Aspose.Cells for .NET provides a powerful and flexible solution for developers. In this guide, we covered:
- Basic and advanced Excel-to-PDF conversion techniques.
- Compliance and tracking options for enhanced control.
For more details, refer to the documentation or contact us on our forum.