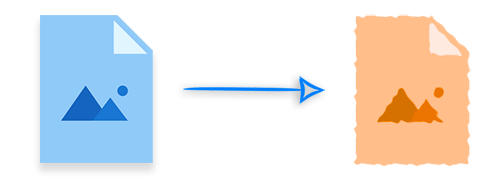
Image editing is a vital component in many applications, allowing for the enhancement and manipulation of images for further processing. In our previous blogs, we covered various topics, including cropping images, rotating images, and blurring images. Today, we will explore how to apply median and wiener filters—two essential techniques for denoising and smoothing images. Let’s dive into how to apply median and wiener filters to an image programmatically in C#.
- C# API to Apply Median and Wiener Image Filters
- Apply Median Filter to an Image in C#
- Apply Gauss Wiener Filter to an Image
- Motion Wiener Filtering of an Image
C# API to Apply Median and Wiener Image Filters - Free Download
Aspose.Imaging for .NET is a robust API tailored for implementing image editing features in .NET applications. We will utilize this API to apply median and wiener filters on images. You can easily download the API or install it directly from NuGet with the following command:
PM> Install-Package Aspose.Imaging
Apply Median Filter to an Image in C#
The median filter is a nonlinear digital filtering technique that effectively reduces noise in images, making it an essential tool in C# image processing. Here’s how to apply a median filter to an image in C#:
- Load the image using the Image.Load() method.
- Cast the image to the RasterImage type.
- Create an instance of the MedianFilterOptions class and initialize it with the desired rectangle size.
- Apply the median filter using the RasterImage.Filter(Rectangle, MedianFilterOptions) method.
- Save the resultant image using the RasterImage.Save() method.
Here’s a code sample demonstrating how to apply a median filter to an image in C#:
Below is the image before and after applying the median filter:
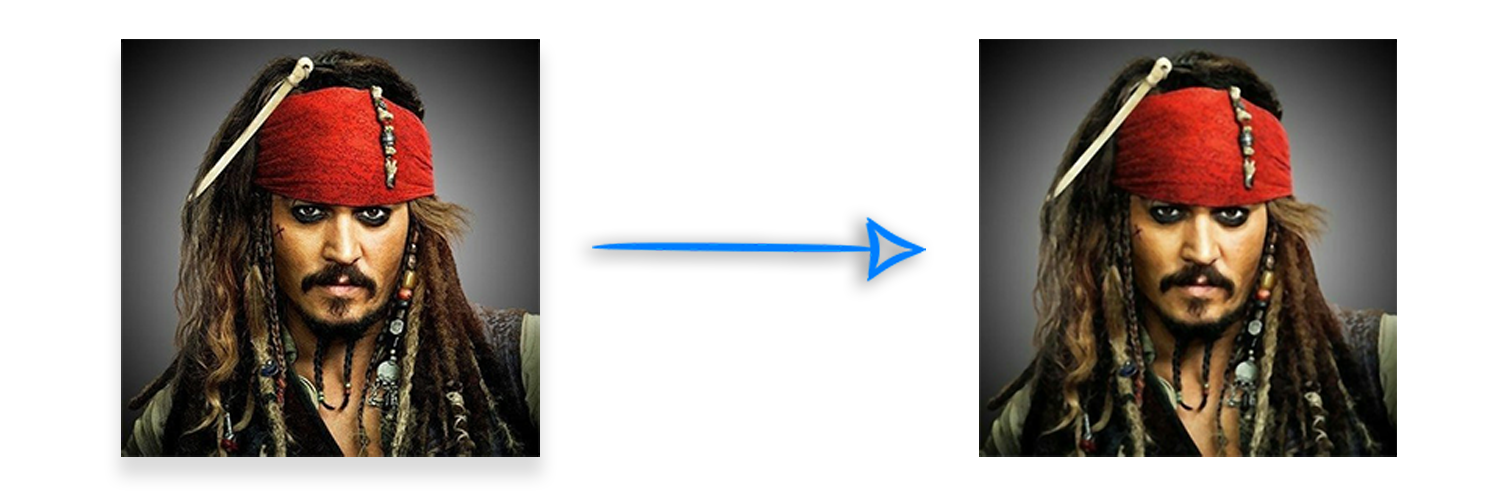
Apply Gauss Wiener Filter to an Image in C#
The Gauss Wiener filter is another effective method for minimizing additive noise and blurring in images. Follow these steps to apply a Gauss Wiener filter in C#:
- Load the image using the Image.Load() method.
- Cast the image to the RasterImage type.
- Create an instance of the GaussWienerFilterOptions class and initialize it with the desired radius size and smooth value.
- (Optional) For a grayscale image, set the GaussWienerFilterOptions.Grayscale property to true.
- Apply the Gauss Wiener filter using the RasterImage.Filter(Rectangle, GaussWienerFilterOptions) method.
- Save the resultant image using the RasterImage.Save() method.
Here’s a code sample for applying a Gauss Wiener filter to an image in C#:
Below you can see the image before and after applying the Gauss Wiener filter with the grayscale option:
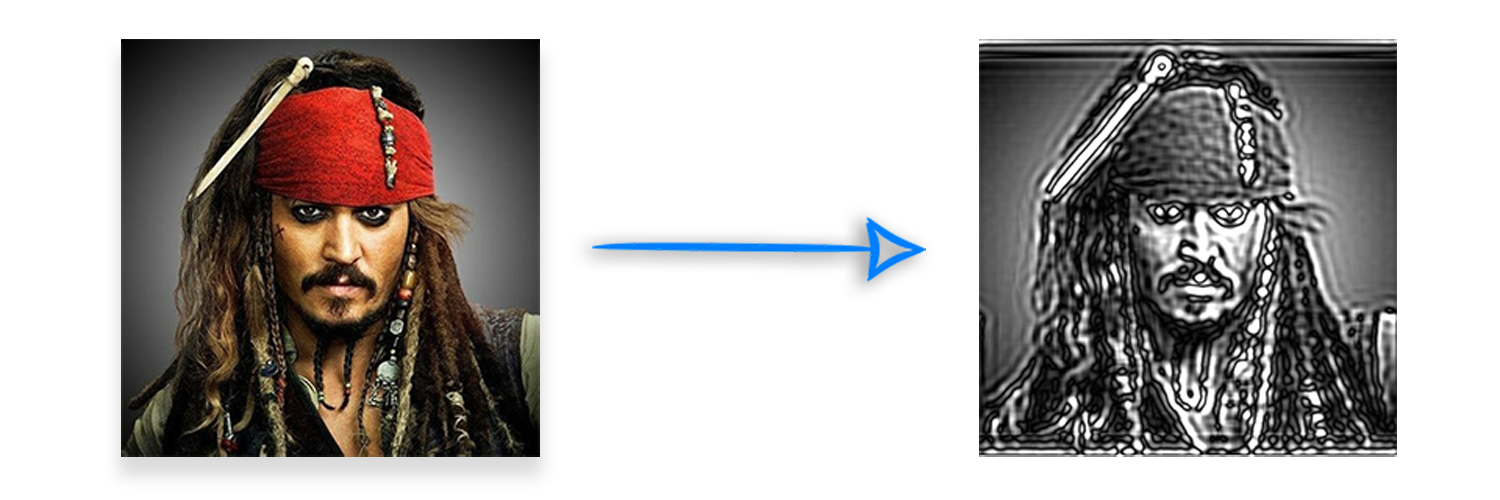
And here’s the image before and after applying the Gauss Wiener filter without grayscale:

Apply Motion Wiener Filter to an Image in C#
The motion Wiener filter is specifically designed to eliminate blurring caused by moving objects. Here’s how to apply the motion Wiener filter in C#:
- Load the image using the Image.Load() method.
- Cast the image to the RasterImage type.
- Create an instance of the MotionWienerFilterOptions class and initialize it with the length, smooth value, and angle.
- Apply the motion Wiener filter using the RasterImage.Filter(Rectangle, MotionWienerFilterOptions) method.
- Save the resultant image using the RasterImage.Save() method.
Here’s a code sample showing how to apply a motion Wiener filter to an image in C#:
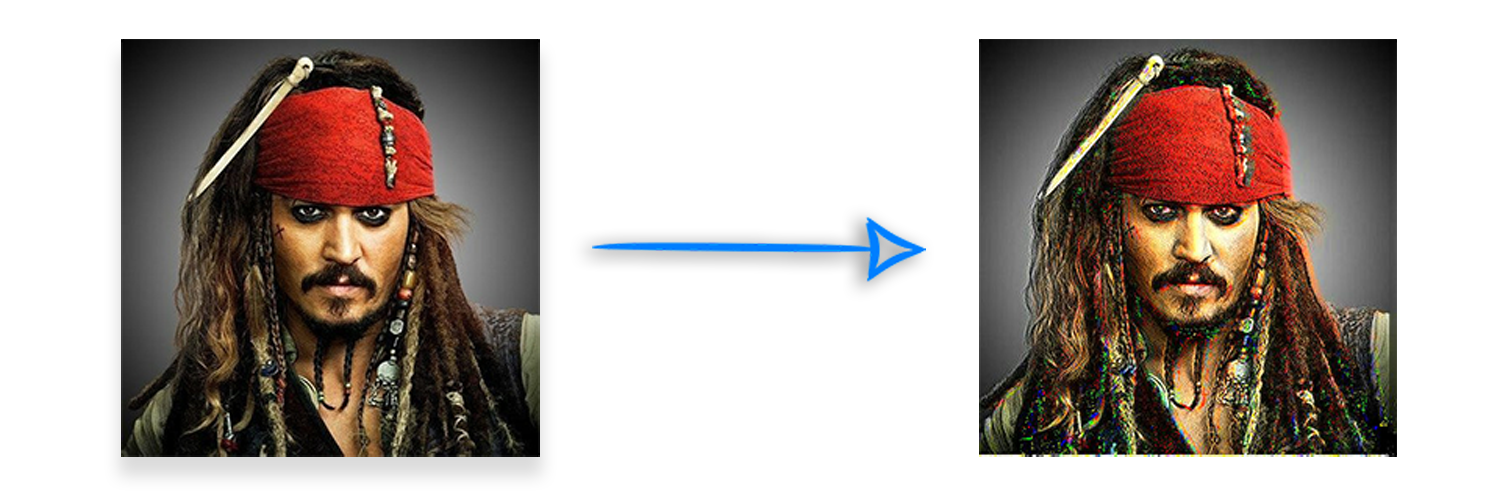
C# Median and Wiener Image Filtering API - Get a Free License
You can get a free temporary license to apply median and wiener filters to images without any evaluation limitations.
Conclusion
In this article, we explored how to apply median and wiener filters to images in C#. We also discussed methods to reduce noise from moving objects in images. You can seamlessly integrate these features into your C# applications to enhance your image editing capabilities. Whether you’re looking for a C# image processing tutorial for beginners or seeking advanced techniques, the Aspose Plugin offers a comprehensive solution for all your image processing needs.
Read More
To learn more about the .NET image processing API, check out the documentation. If you have any questions, feel free to reach out through our forum.