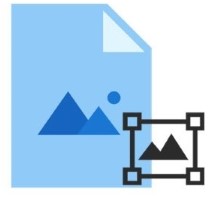
Watermarks are essential for claiming ownership of images and preventing unauthorized usage or counterfeiting. In many scenarios, you may need to implement an automated watermarking feature in your web or desktop applications. This article will guide you through adding watermarks to images programmatically using C#. You’ll also learn how to watermark a batch of images efficiently. Let’s get started!
Table of Contents
- C# API to Add Watermark to Images
- Add Watermark to an Image in C#
- Add Diagonal Watermark to Images in C#
- Get Free API License
Add Watermark to Images in C# - API Installation
To add watermarks to images, we will utilize the Aspose.Imaging for .NET. This powerful image-processing API supports a variety of image formats and simplifies image manipulation. You can either download the API binaries or install it via NuGet using the following command:
PM> Install-Package Aspose.Imaging
Add Watermark to an Image in C#
Here’s a step-by-step guide on how to add a watermark to an image using C#:
- Load the image using the Image class.
- Create an instance of the Graphics class, initializing it with the Image object.
- Define the font family, size, and style using the Font class.
- Create a SolidBrush instance to set properties like color.
- Instantiate the StringFormat class to manage text alignment.
- Add the watermark to the image using the Graphics.DrawString(String, Font, SolidBrush, 0, 0, StringFormat) method.
- Save the image using the Image.Save(String) method.
Here’s a code sample demonstrating how to add a watermark to an image in C#:
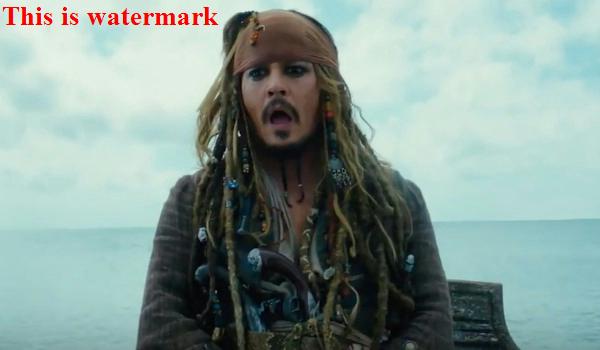
C# Add Diagonal Watermark to Images
In some cases, watermarks are applied diagonally across images. The Aspose.Imaging for .NET API allows you to rotate the watermark text to achieve this effect. Follow these steps to add a diagonal watermark:
- Load the image using the Image class.
- Create a Graphics instance initialized with the Image object.
- Define the font family, size, and style with the Font class.
- Create a SolidBrush instance to set properties like color.
- Instantiate a StringFormat object for text alignment.
- Create a Matrix instance to set the transformation angle.
- Assign the Matrix object to the Graphics.Transform property.
- Add the watermark using the Graphics.DrawString(String, Font, SolidBrush, 0, 0, StringFormat) method.
- Save the image using the Image.Save(String) method.
Here’s a code sample that illustrates how to add a diagonal watermark to images using C#:
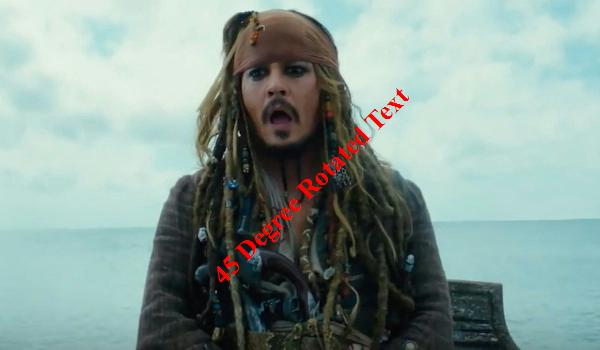
Insert Watermark on Images with a Free License
You can get a free temporary license to insert watermarks on images without any evaluation limitations.
Conclusion
In this article, you learned how to add a watermark to images in C#. You also discovered how to rotate watermark text to a specific angle. For further information about the C# image processing API, please refer to the documentation. If you have any questions or need assistance, feel free to reach out via our forum.