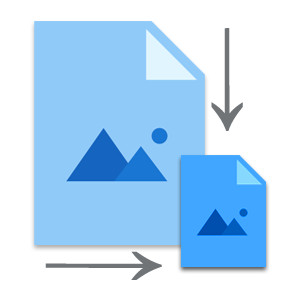
Aspose.Imaging’s Image Resizer Plugin for .NET simplifies the process of batch image resizing and raster image resizing while maintaining high-quality output. Whether you’re developing .NET Core image resizing applications for the web or optimizing images for mobile, this plugin offers seamless integration into your .NET workflows. In this article, we’ll explore how to programmatically resize images in C#, focusing on raster and vector formats, as well as best practices for image scaling.
Topics Covered:
- C# Image Resizing for Web Applications
- Batch Image Resizing in C#
- Proportional Image Resizing
- Vector Image Resizing in .NET
C# Image Resizing for Web Applications
To resize images, we will use Aspose.Imaging for .NET, a powerful image manipulation library that supports various raster and vector formats, easily integrated into .NET web applications. The API can be installed via NuGet, allowing for quick setup in your projects.
PM> Install-Package Aspose.Imaging
Batch Image Resizing in C#
In .NET, two methods can be utilized for efficient image resizing: simple resizing and resizing using a specified resize type. With batch image resizing, you can process multiple images simultaneously, optimizing time and resources. Below are steps to accomplish resizing.
Simple Image Resizing in C#
Follow these steps to resize an image in C#:
- Load the image file using the Image class.
- Resize the image by calling the Image.Resize(Int32, Int32) method.
- Save the resized image using the Image.Save(string) method.
Here’s a sample code demonstrating basic image resizing in a .NET application.
Resize Images Based on Screen Size C#
To resize images in accordance with different device screen sizes, you need to determine the screen resolution and adapt the resizing parameters appropriately. This can ensure high-quality image rendering across devices.
// Pseudo-code for resizing based on screen size
int screenWidth = GetScreenWidth();
int screenHeight = GetScreenHeight();
image.Resize(screenWidth, screenHeight);
Proportional Image Resizing in C#
Instead of specifying fixed height and width—which may distort the image—proportional resizing maintains the aspect ratio. This can be achieved as follows:
- Load the image using the Image class.
- Cache the image data using the Image.CacheData() method.
- Specify new dimensions while respecting the original aspect ratio.
- Save the resized image using the Image.Save(string) method.
Example code for proportional image resizing in C# is as follows:
Vector Image Resizing in .NET
Aspose.Imaging for .NET allows you to resize vector images like SVG and save them in raster formats. Below is a sample demonstrating how to resize an SVG image and save it in PNG format:
C# Image Resizing with a Free License
You can get a free temporary license for Aspose.Imaging to resize images without any evaluation limitations.
Conclusion
In this article, you have learned effective techniques for batch image resizing and raster image resizing programmatically in C#. The provided code samples illustrate both simple and proportional resizing methods, ensuring quality retention. We also covered resizing vector images and handling aspect ratios without third-party tools. For further exploration, refer to the documentation for more on the .NET image processing API. You can also download a package of example source code from GitHub. For any inquiries, feel free to reach out on our forum.