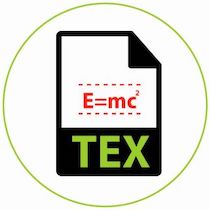
LaTeX는 수학적 내용을 조판하는 강력한 도구로, TEX 파일 내에 공식을 포함할 수 있습니다. LaTeX에서 준비한 이 소스 문서는 텍스트, 기호, 수학 표현 및 그래픽을 형식화하는 다양한 명령을 제공합니다. 이 기사에서는 C#을 사용하여 LaTeX 방정식 및 수학 공식을 효율적으로 렌더링하는 방법을 알아볼 것입니다.
목차
- C# API로 LaTeX 방정식 및 수학 공식 렌더링
- C#을 사용하여 LaTeX 인라인 수학 공식 렌더링
- 복잡한 방정식 렌더링
- 긴 방정식 표시
- 여러 방정식 정렬
- 방정식 그룹화 및 중앙 정렬
- 행렬, 괄호 및 대괄호 렌더링
- 분수 및 이항 렌더링
C# API로 LaTeX 방정식 및 수학 공식 렌더링
LaTeX 수학 공식을 렌더링하기 위해 Aspose.TeX for .NET API를 활용할 것입니다. 이 강력한 .NET LaTeX 방정식 렌더링 라이브러리는 TeX 파일을 PDF, XPS 또는 이미지와 같은 다양한 형식으로 조판할 수 있습니다.
API의 DLL을 다운로드하거나 NuGet을 사용하여 설치할 수 있습니다:
PM> Install-Package Aspose.TeX
C#을 사용하여 LaTeX 인라인 수학 공식 렌더링
간단한 인라인 수학 공식이나 방정식을 렌더링하는 것은 간단합니다. 다음 단계를 따르세요:
- MathRendererOptions 클래스의 인스턴스를 생성합니다.
- LaTeX 문서의 서문을 지정합니다.
- 선택적으로 Scale, TextColor, BackgroundColor 등의 속성을 조정합니다.
- 공식 이미지의 출력 스트림을 생성합니다.
- Render() 메서드를 호출하여 공식을 렌더링하고, 공식 문자열, 스트림, MathRendererOptions 및 출력 이미지 크기를 인수로 전달합니다.
다음은 C#을 사용하여 수학 공식을 프로그래밍 방식으로 렌더링하는 방법을 보여주는 코드 샘플입니다:

C#을 사용하여 인라인 수학 공식 렌더링
C#에서 복잡한 LaTeX 방정식 렌더링
복잡한 LaTeX 방정식을 렌더링하려면 이전과 동일한 단계를 따르되, 4단계에서 더 복잡한 공식 문자열을 사용합니다:
MathRenderer.Render(@"\begin{equation*}
e^x = x^{\color{red}0} + x^{\color{red}1} +
\frac{x^{\color{red}2}}{2} +
\frac{x^{\color{red}3}}{6} +
\cdots = \sum_{n\geq 0} \frac{x^{\color{red}n}}{n!}
\end{equation*}", stream, options, out size);

C#에서 복잡한 방정식 렌더링
C#에서 긴 방정식 표시
여러 줄에 걸쳐 긴 방정식을 표시하려면 다음 접근 방식을 사용하세요:
MathRenderer.Render(@"\begin{document}
\begin{multline*}
p(x) = x^1+x^2+x^3+x^4\\
- x^4 - x^3 - x^2 - x
\end{multline*}
\end{document}", stream, options, out size);

C#에서 긴 방정식 표시
C#을 사용하여 여러 방정식 정렬
4단계에서 공식 문자열을 수정하여 여러 방정식을 정렬할 수 있습니다:
MathRenderer.Render(@"\begin{document}
\begin{align*}
a+b & a-b & (a+b)(a-b)\\
x+y & x-y & (x+y)(x-y)\\
p+q & p-q & (p+q)(p-q)
\end{align*}
\end{document}", stream, options, out size);
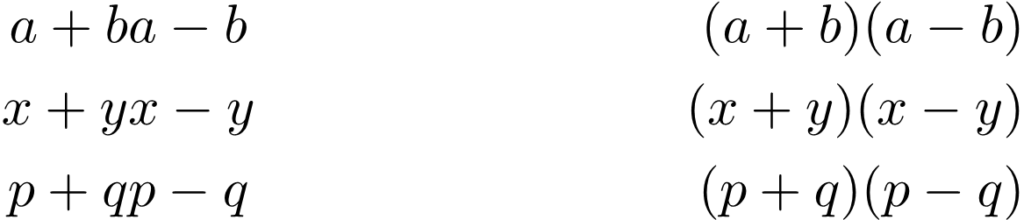
C#을 사용하여 여러 방정식 정렬
C#을 사용하여 방정식 그룹화 및 중앙 정렬
여러 방정식을 그룹화하고 중앙 정렬하려면 다음 코드를 사용하세요:
MathRenderer.Render(@"\begin{gather*}
(a+b)=a^2+b^2+2ab \\
(a-b)=a^2+b^2-2ab \\
(a-b)=a^2+b^2-2ab
\end{gather*}", stream, options, out size);
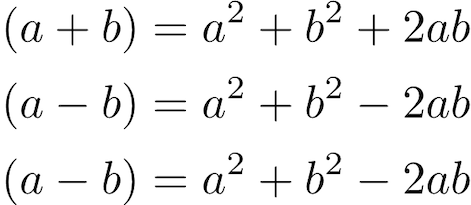
C#을 사용하여 방정식 그룹화 및 중앙 정렬
C#에서 행렬, 괄호 및 대괄호 렌더링 {#Render-Matrices,-Parenthesis,-and-Brackets-in-CSharp}
행렬, 괄호 및 대괄호를 렌더링하는 것도 유사하게 수행할 수 있습니다:
MathRenderer.Render(@"\begin{document}
[
\left \{
\begin{tabular}{ccc}
1 & 4 & 7 \\
2 & 5 & 8 \\
3 & 6 & 9
\end{tabular}
\right \}
]
\end{document}", stream, options, out size);
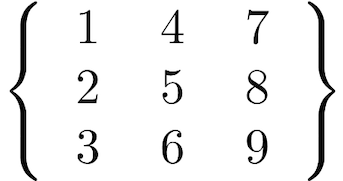
C#에서 행렬, 괄호 및 대괄호 렌더링
C#을 사용하여 분수 및 이항 렌더링
분수 및 이항을 렌더링하려면 다음 구조를 따르세요:
MathRenderer.Render(@"\begin{document}
[
\binom{n}{k} = \frac{n!}{k!(n-k)!}
]
\end{document}", stream, options, out size);
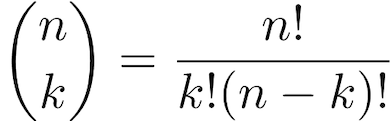
C#을 사용하여 분수 및 이항 렌더링
무료 라이센스 받기
제한 없이 라이브러리를 평가할 수 있는 무료 임시 라이센스를 받을 수 있습니다.
결론
이 기사에서는 다음을 탐구했습니다:
- C#에서 간단하고 복잡한 수학 공식 및 방정식을 렌더링하는 방법.
- 프로그래밍 방식으로 방정식을 정렬하고 그룹화하는 방법.
- 행렬, 괄호, 대괄호, 분수 및 이항을 렌더링하는 방법.
C#에서 LaTeX 방정식 및 수학 공식을 렌더링하는 것 외에도 문서를 참조하여 Aspose.TeX for .NET API에 대해 더 깊이 알아볼 수 있습니다. 질문이 있으시면 무료 지원 포럼에서 문의해 주세요.