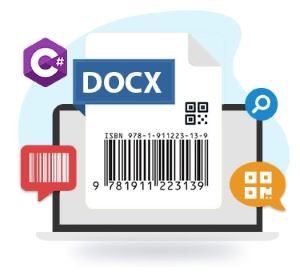
Barcodes are images created from parallel lines, dots, or rectangles that encode data/information. Industry professionals use barcodes to embed product information, track product movement, and manage inventory. In certain scenarios, we might need to add dynamic barcode to Word document C#. Microsoft Word, a widely used graphical word processing program, supports the popular file formats DOCX and DOC. In this article, we will cover how to create barcode in Word document using C# Aspose.
The following topics will be addressed:
- C# API to Create Barcode in Word Documents
- Generate and Add Barcode to Word Document
- Add Barcode to Existing Word Document
- Add QR Code to Word Document
- Read Barcode from Word Document
C# API to Create Barcode in Word Documents
To read barcode data from Word document C# ASP.NET MVC, we will follow a two-step approach. First, the Aspose.Words for .NET API will be used to create or load a Word document. Then, we will generate the barcode image using the Aspose.BarCode for .NET API. The Document class of the Aspose.Words API enables the creation of new Word documents or loading existing ones. The Save() method of this class allows us to save the document at a specified file path. The DocumentBuilder class provides methods for building documents, including InsertImage() methods to insert images into the document.
The Aspose.BarCode for .NET API supports various types of supported barcodes. To generate barcodes, it provides the BarcodeGenerator class that requires EncodeType and text to encode as parameters. The generated barcode can be saved using the Save() method. Additionally, the API offers the BarCodeImageFormat enumeration for specifying save formats, and the BarCodeReader class is available for reading barcodes from images.
Please either download the DLLs of the APIs or install them using NuGet.
PM> Install-Package Aspose.BarCode
PM> Install-Package Aspose.Words
Generate and Add Barcode to Word Document in C#
To generate barcode in Word C# .NET, follow these steps:
- Create an instance of the BarcodeGenerator class with the EncodeType and text to encode as arguments.
- Instantiate a memory stream object.
- Call the Save() method to save the barcode image to the memory stream.
- Create a new instance of the Document class.
- Initialize a DocumentBuilder instance with the Document object.
- Use the InsertImage() method to insert the barcode image using the memory stream.
- Finally, call the Save() method, specifying the output DOCX file path.
The following code example demonstrates how to generate barcode in Word document C# .NET efficiently:
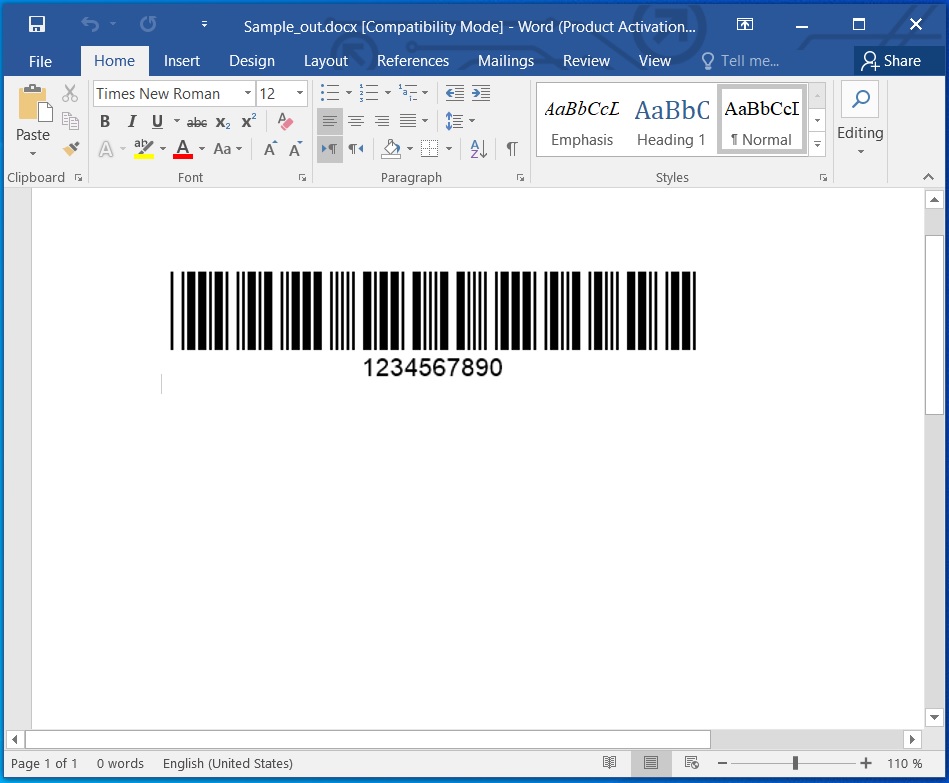
Generate and add Barcode to a new Word document in C#.
Add Barcode to Existing Word Document in C#
To add barcode to existing Word document using C#, follow these steps:
- Instantiate the BarcodeGenerator class, providing the EncodeType and text to encode.
- Create a memory stream object.
- Save the barcode image to the memory stream using Save().
- Load the existing Word document with the Document class.
- Initialize a DocumentBuilder instance with the Document object.
- Insert the barcode image using InsertImage() method and the memory stream.
- Call the Save() method with the desired DOCX file path.
The following code example shows create barcode in Word document with C# .NET:
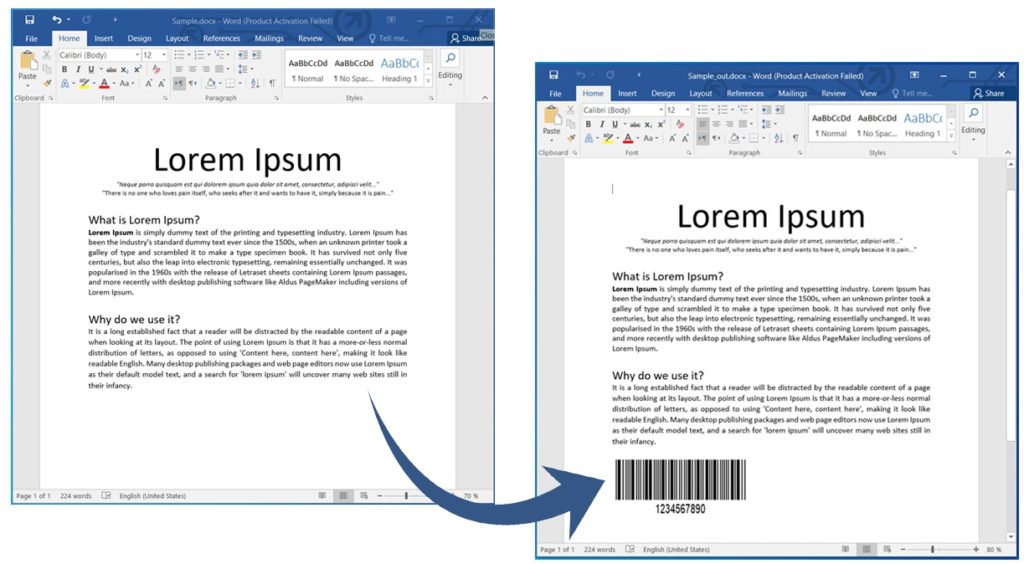
Add Barcode to Existing Word Document in C#.
Add QR Code to Word Document using C#
Similarly, to add a QR code image to a Word document:
- Create an instance of the BarcodeGenerator class and set the EncodeType to QR or GS1QR.
- Follow the same steps as above to save and insert the image.
The following example illustrates how to add QR code to Word document using C# .NET:
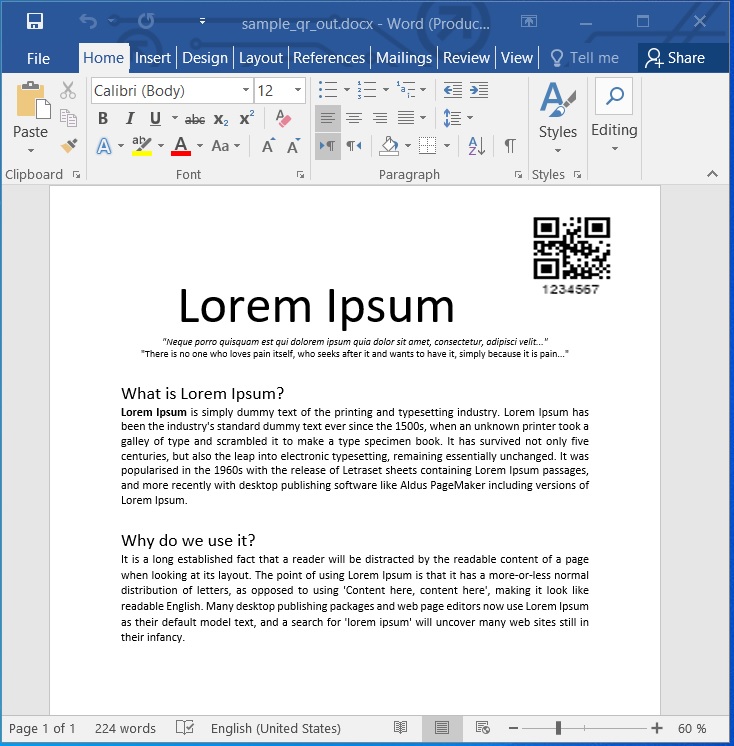
Add QR Code to Word Document using C#.
Read Barcode from Word Document using C#
To read barcode from Word document C#:
- Load the existing Word document with the Document class.
- Access the NodeCollection of Shape types using the GetChildNodes() method.
- Loop through the shapes and check if the shape is an image.
- Save the image to the stream.
- Create a BarCodeReader instance with the image stream and DecodeType parameters.
- Call ReadBarCodes() method to retrieve BarCodeResult.
- Display the barcode information.
The following code example demonstrates how to read barcode data from Word document using Aspose C#.
Codetext found: 1234567890, Symbology: Code39Standard
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have covered:
- How to create a Word document programmatically.
- Steps to generate a barcode image and add it to the Word document.
- How to create a QR code and insert it into a Word document.
- Techniques to read a barcode image from a Word document in C#.
For further information, refer to the Aspose.BarCode for .NET API documentation. If you have any questions, please feel free to reach out on the forum.