This article covers various approaches to find and replace text in Word documents using C# in .NET or .NET Core applications, leveraging the Aspose.Words library for efficient programmatic Word document editing and automation.
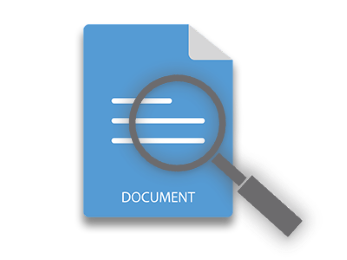
Find and Replace is an essential feature of MS Word, allowing users to quickly locate and replace desired text in documents. It becomes increasingly convenient and time-efficient when dealing with lengthy documents. Particularly in scenarios where you may need to replace text in multiple Word documents or automate this process, effective programmatic document manipulation offers the best results. In this article, I will demonstrate how to find and replace text in Word documents programmatically using C#.
Once you read this article, you will be capable of:
- find and replace specific text in Word document C#
- find and replace similar words in Word document
- find and replace text using Regex
- find and replace text in the header/footer of Word document
- find and replace text with meta-characters in Word document
C# Library to Find and Replace Text in a Word Document
First, create a new C# project (Console, ASP.NET, etc.) in Visual Studio and install the Aspose.Words for .NET library using NuGet Package Manager or Package Manager Console.
Installing via NuGet Package Manager
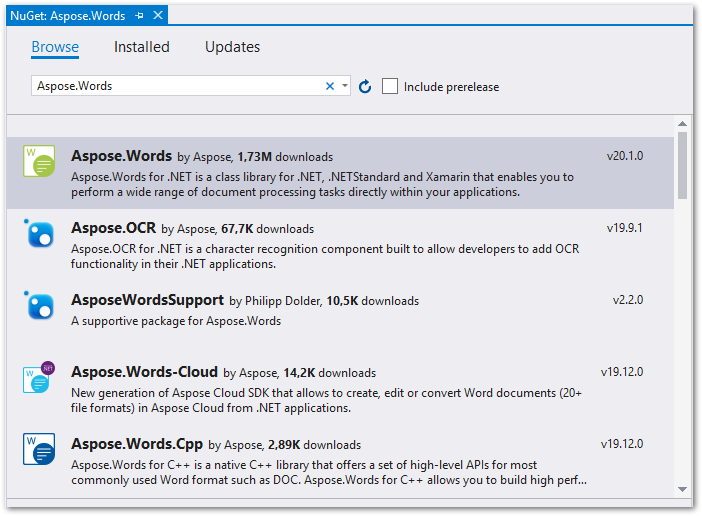
Installing via Package Manager Console
PM> Install-Package Aspose.Words
After we have installed Aspose.Words for .NET, let’s begin with finding and replacing text in the following Word document.
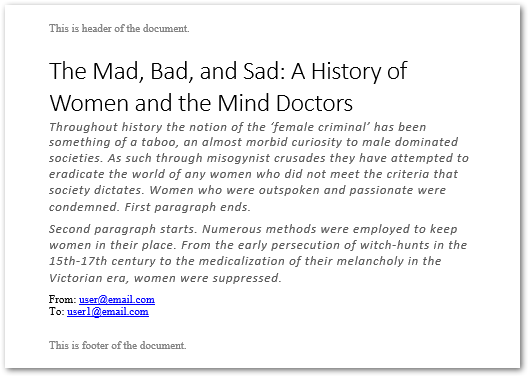
Find and Replace Text in Word Documents in C#
The following steps outline how to find and replace text in a Word document using the Aspose.Words for .NET library:
- Create an instance of the Document class and initialize it with the Word document’s path.
- Use the Document.Range.Replace(string, string, FindReplaceOptions) method to perform the C# find and replace Word document operation.
- Save the modified document using Document.Save(string).
The FindReplaceOptions class provides various options to customize the find/replace operations. The following code sample illustrates how to find and replace a specific word or string in a Word document using C#.
Output
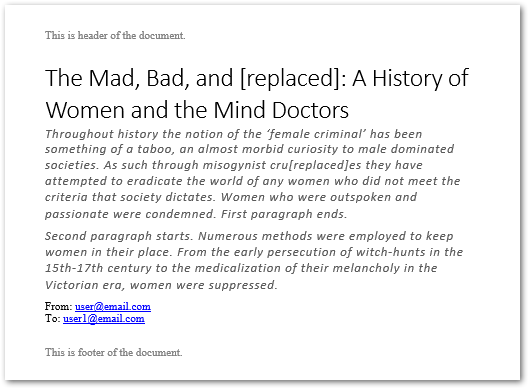
Find and Replace Similar Words in Word Documents using C#
You can customize the Aspose.Words API to find similar words and replace them with a specific term. For instance, you might want to find the words “sad” and “mad” and replace them with a single word. The following code sample demonstrates how to find and replace similar words in a Word document using C#.
Output
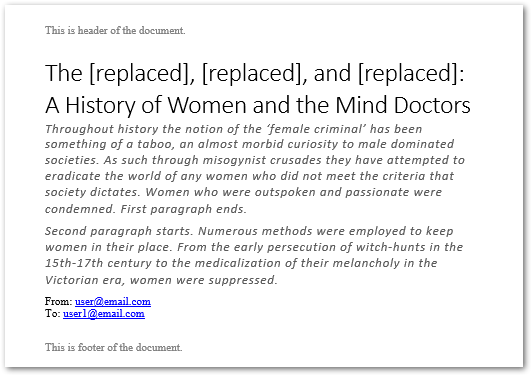
Find and Replace Text using Regex in C#
In some cases, you may need to find and replace text that matches a particular pattern, such as hiding or replacing all email IDs in a Word document. You can create a regular expression for email IDs and utilize it with the Document.Range.Replace(Regex, string, FindReplaceOptions) method.
The following code sample illustrates how to find and replace text in a Word document based on a regex pattern.
Output
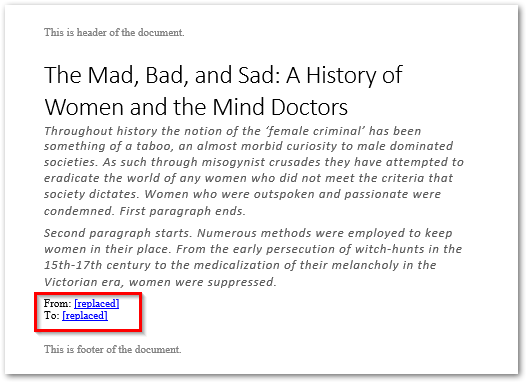
Find and Replace Text in Header/Footer of Word Document using C#
You can also find and replace text in the header or footer sections of a Word document using the HeaderFooter class. The HeaderFooter.Range.Replace(string, string, FindReplaceOptions)
method can be utilized for this purpose. The following code sample shows how to replace text in the header/footer of a Word document in C#.
Output
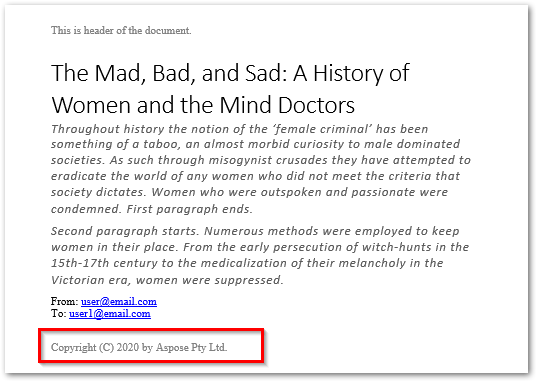
Find and Replace Text with Meta-Characters in Word Documents using C#
In scenarios where the text or phrase spans multiple paragraphs, sections, or pages, the basic find and replace methods may not suffice. You can utilize Aspose.Words’ meta-characters to facilitate this:
- &p: paragraph break
- &b: section break
- &m: page break
- &l: line break
The following code sample illustrates how to find and replace text with a paragraph break in a Word document.
Output
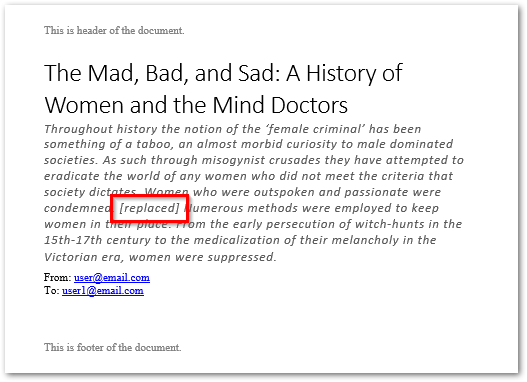
Conclusion
This article presents several approaches to find and replace text in a Word document based on matched or similar words, phrases, and regex patterns programmatically. These features not only streamline the text replacement process but also significantly reduce the time and effort needed for manual find and replace operations in Word documents. For further insights into programmatic Word document editing with .NET, I encourage you to explore Aspose’s comprehensive documentation.
Related Article(s)
- Find and Replace Text in Word Documents using Java
- .NET Word Automation - Create Rich Word Documents in C#
Tip: You may also find a free Text to GIF Converter useful for generating animations from texts.